Sorting with index in JavaScript
Published: 2023-09-14 09:23:03
How to Sort an Array with Index in JavaScript
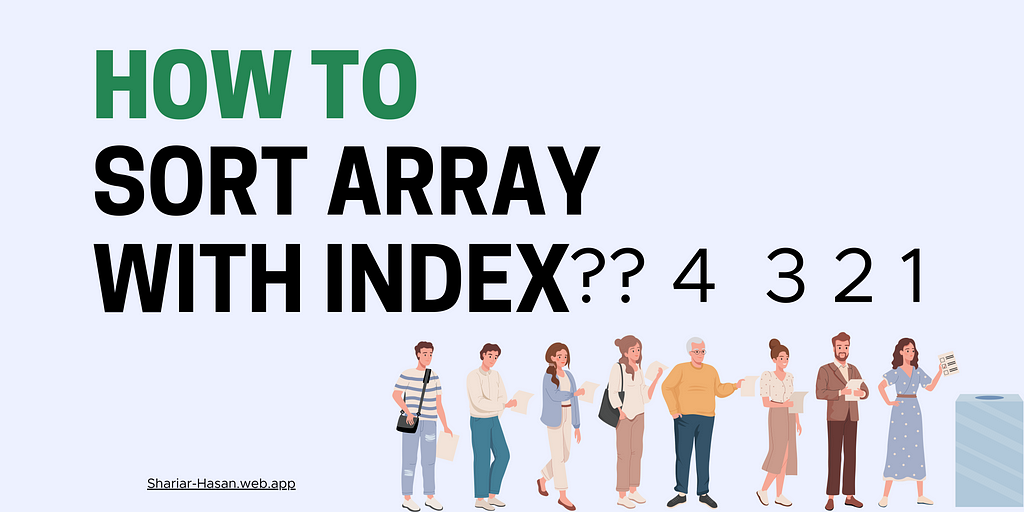
Assalamu Alaikum,
Sorting arrays in JavaScript is a common task, and the built-in sort( ) method does a great job of sorting the elements in an array. However, there are situations where you need to sort an array while keeping track of the original indices of the elements. This can be useful when you want to reorder another associative item based on the sorted array. In this article, we'll explore how to sort an array with index in JavaScript.
Why sorting with index?
Consider an array of objects where each object represents a person with properties like name and age . You want to sort this array based on the age property but also keep track of the original order to display the sorted list with associated data later. The sort( ) method alone won't be enough because it only sorts the elements without preserving the original indices.
How to do this?
To sort an array with an index in JavaScript, you can follow these steps:
- Create an array of index-value pairs.
- Sort the index-value pairs based on the requirement.
- Use the sorted values the original array based on the sorted indices.
Lets see Codes
// Sample array of objects
const people = [
{ name: "Alice", age: 32 },
{ name: "Bob", age: 28 },
{ name: "Charlie", age: 45 },
];
// Step 1: Create an array of index-value pairs
const indexedArray = people.map((item, index) => ({ index, value: item }));
// Step 2: Sort the index-value pairs based on the requirement (based on age)
indexedArray.sort((a, b) => a.value.age - b.value.age);
// Step 3: Use the sorted values the original array based on the sorted indices
const sortedPeople = indexedArray.map((item) => item.value);
// Now, 'sortedPeople' contains the sorted array of objects by age
// and indexed array will contain the track of indices
console.log(sortedPeople);
Sorting an array with an index allows you to maintain the original order of elements while sorting them according to your specific requirements. Whether you’re working with complex data structures or simple arrays, this approach can help you solve various sorting challenges in your JavaScript projects.
Happy Reading ! 🥳 Allah Hafez.
sortingindexjavascript
Published: 2023-09-14 09:23:03