Capitalizing Strings in JavaScript: Different Methods
Published: 2023-09-09 18:48:15
Different methods on capitalizing a string
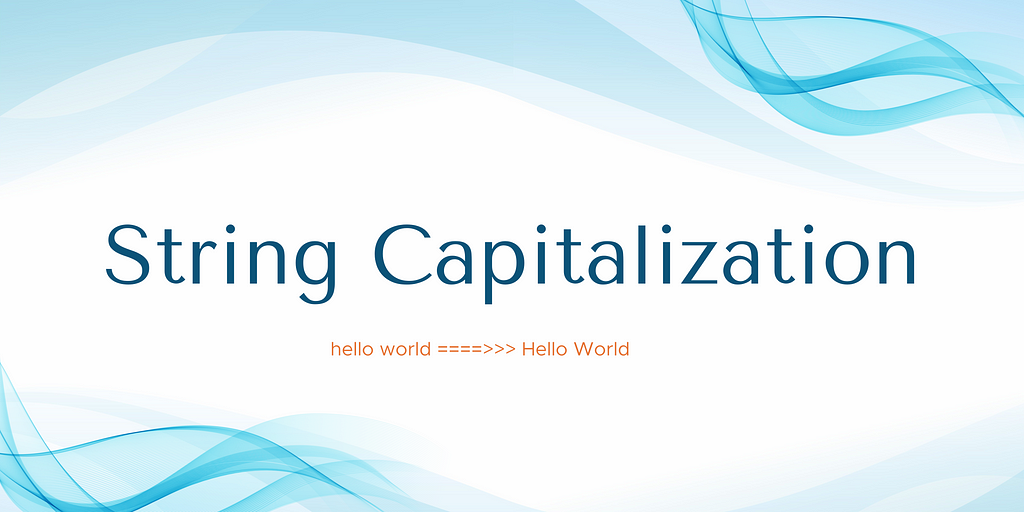
When working with strings in JavaScript, many of us might come across scenarios where we need to capitalize the first letter of each word or the entire string. Javascript has in built methods of make the full screen uppercase or lowercase, but does not have any capitalize method. In this article, we’ll explore various methods to capitalize strings in JavaScript.
1. Using the toUpperCase and toLowerCase Methods
One of the simplest ways to capitalize a string is by using the toUpperCase and toLowerCase methods along with string manipulation. Here's an example:
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase();
}
const inputString = "hello, world!";
const capitalizedString = capitalizeFirstLetter(inputString);
console.log(capitalizedString);
// Output: "Hello, world!"
In this function, we capitalize the first letter using toUpperCase() and make the rest of the string lowercase with toLowerCase(). This method works well for capitalizing the first letter of a sentence or title case.
2. Using Regular Expressions
Regular expressions can be powerful tools for manipulating strings. You can capitalize the first letter of each word in a string using regular expressions in JavaScript:
function capitalizeWords(string) {
return string.replace(/\b\w/g, char => char.toUpperCase());
}
const inputString = "hello, world!";
const capitalizedString = capitalizeWords(inputString);
console.log(capitalizedString);
// Output: "Hello, World!"
In this function, the regular expression \b\w matches the first letter of each word (where \b denotes a word boundary and \w matches a word character). We then use the replace method to replace these matches with their uppercase versions.
3. Using the split and join Methods
You can also capitalize the first letter of each word by splitting the string into an array of words, capitalizing each word, and then joining them back together:
function capitalizeWords(string) {
const words = string.split(' ');
const capitalizedWords = words.map(word => word.charAt(0).toUpperCase() + word.slice(1));
return capitalizedWords.join(' ');
}
const inputString = "hello, welcome to world!";
const capitalizedString = capitalizeWords(inputString);
console.log(capitalizedString);
// Output: "Hello, Welcome To World!"
This method provides more flexibility if you need to manipulate individual words in a sentence.
Conclusion
Capitalizing strings in JavaScript is a common task, and there are various methods to achieve it. Depending on your specific use case, you can choose the method that suits you best. Whether you prefer simple string manipulation, regular expressions, split and joint etc. these techniques will help you present your text in a well written and professional manner.
capitalizationstring-manipulation
Published: 2023-09-09 18:48:15