Check empty object in JavaScript.
Published: 2023-05-24 18:50:46
Check empty object in JavaScript
Different Ways to Check If an Object is Empty in JavaScript
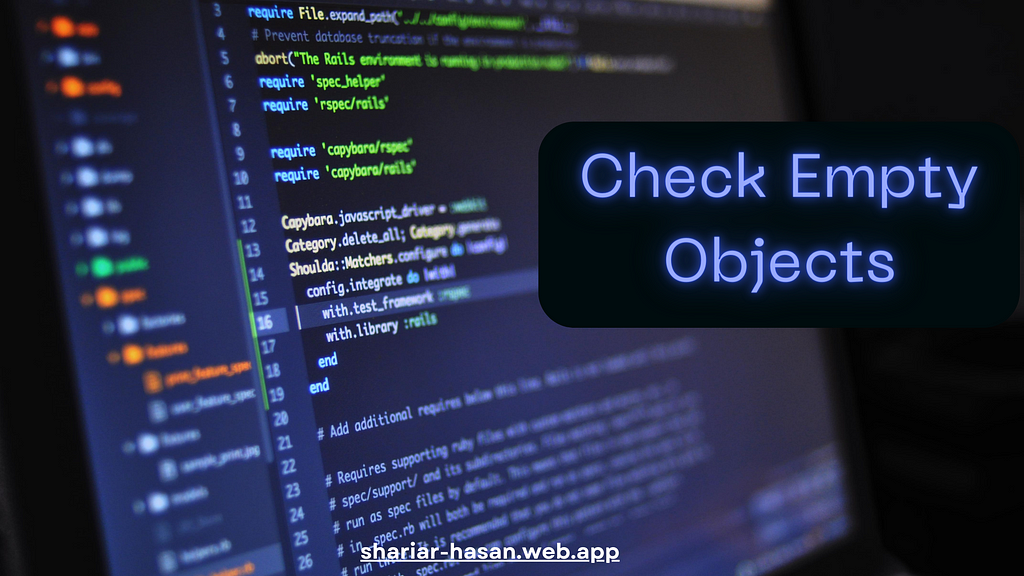
In our JavaScript journey, there's many times we have to check whether an object is empty or not. Well, an empty object mainly means an object that does not have any key-value properties. In this blog, we will learn some of the methods for checking whether a JavaScript object is empty or not.
Method 1: Using the Object.keys() Method:
Basically, the Object.keys() method returns an array, that contains all the key values. if we check the length of this array, we will know if the object is empty or not (as an empty object returns length 0).
const yourObj = {};
console.log(Object.keys(yourObj).length === 0); // true
const anotherObj = {
name : "Medium"
};
console.log(Object.keys(anotherObj).length === 0); // false
Method 2: Using the JSON.stringify() Method
Basically, the JSON.stringify() method converts a JavaScript object into a string. By using this, we can compare the string “{ }” with our Stringfield object to know if the object is empty or not.
const yourObj = {};
console.log(JSON.stringify(yourObj) === "{}"); // true
const anotherObj = {
name : "Medium"
};
console.log(JSON.stringify(anotherObj) === "{}"); // false
Method 3: Using a for in Loop
Basically, in a for..in loop, we get to iterate over the whole object. If a for loop gets to iterate over any object, that means the object is not empty. So, we will utilize this to check if an object is empty or not.
const isEmptyObject = (obj) => {
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
return false; // if the for loop execute at least one time, the object is not empty
}
}
return true;
}
const yourObj = {};
console.log(isEmptyObject(yourObj)); // true
const anotherObj = {
name : "Medium",
age : 20
};
console.log(isEmptyObject(anotherObj)); // false
These are some of the methods I can use to check if the object is empty or not.
Checking whether an object's is empty or not is a common task in a JavaScript developer's life. The above 3 methods will improve your efficiency, and ensure your code executes with the expected results.
javascriptobjects
Published: 2023-05-24 18:50:46