Building Active Links in Next.js App Router : A Simple Guide
Published: 2024-02-15 20:34:48
Building Active Links in Next.js App Router : A Simple Guide
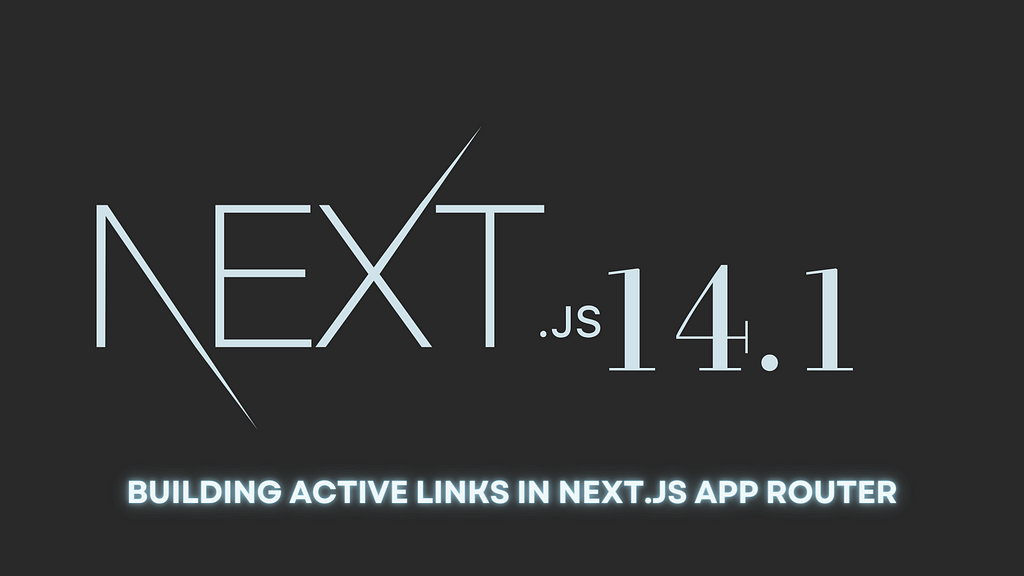
Hey there,Assalamu Alaikum web enthusiasts! 👋 In this post, we’re going to dive into a method for building active links in a Next.js application using React. We’ll create a NavLink component that makes it super easy to highlight the active link in your navigation.
The Problem
When building a web application, you often have a navigation bar with links to different pages. You want the link to look different when you’re on the page it points to. well in React, we use react-router-dom to do it by using react-router-dom’s component. But until the current version of NextJs(14.1) does not provide any active link property/props for the Link component, so we have to design it in custom. Let do that today
The Solution: NavLink Component
"use client";
import Link from "next/link";
import { usePathname } from "next/navigation";
const NavLink = ({
children,
href,
activeClassName,
nonActiveClassName,
className,
...rest
}) => {
const pathname = usePathname(); // p
const isActive = pathname.endsWith(href) || (href.includes(pathname) && pathname !== "/");
const newClassName = `${isActive ? activeClassName : nonActiveClassName} ${className}`;
return (
<Link href={href} className={newClassName} {...rest}>
{children}
</Link>
);
};
export default NavLink;
Explanation
First lets talk about the Props:
- children : React Element/ Jsx Element (Whatever your link text/icons are)
- href : The link that to be routed.
- activeClassName : This is for active link style.
- nonActiveClassName : This is for inactive / opposite of active link style.
- className : Other style class names.
How It Works
Lets get into the main methodology of our approch:
- Checking the Active State: The NavLink component uses the usePathname hook from Next.js to get the current page's pathname.
- Determining the Active Link: It then checks if the current page’s pathname ends with or is included in the provided href. If yes, it marks the link as active or true.
- Styling the Active Link: The component dynamically adds the activeClassName when the link is active and nonActiveClassName when it's not and add them with other className.
Usage Example
// Example Usage
<NavLink
href="/home"
activeClassName="active-link"
nonActiveClassName="inactive-link"
>
Home
</NavLink>
Another Method:
const isActive = pathname.endsWith(href) || (href.startsWith(pathname) && pathname !== "/");
Result
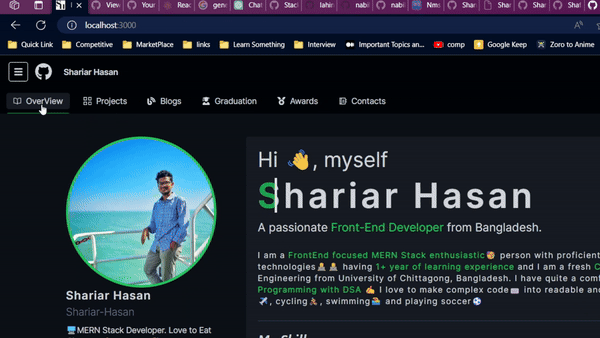
Why This Approach?
- Reusable Component: The NavLink component is reusable across your application. Just drop it in where you need active links!
- Styling: The logic for determining the active link is straightforward, making it easy to understand and customize.
- Integration with Next.js: Using Next.js features like Link and usePathname ensures compatibility and optimization.
Wrapping Up
Creating active links in your Next.js application doesn’t have to be a headache. With that component you can get a clean code setup for active link. Thats it for today.
Allah Hafez.
Happy coding! 🚀
nextjs14nextjs-app-routeractivelinknextjs
Published: 2024-02-15 20:34:48